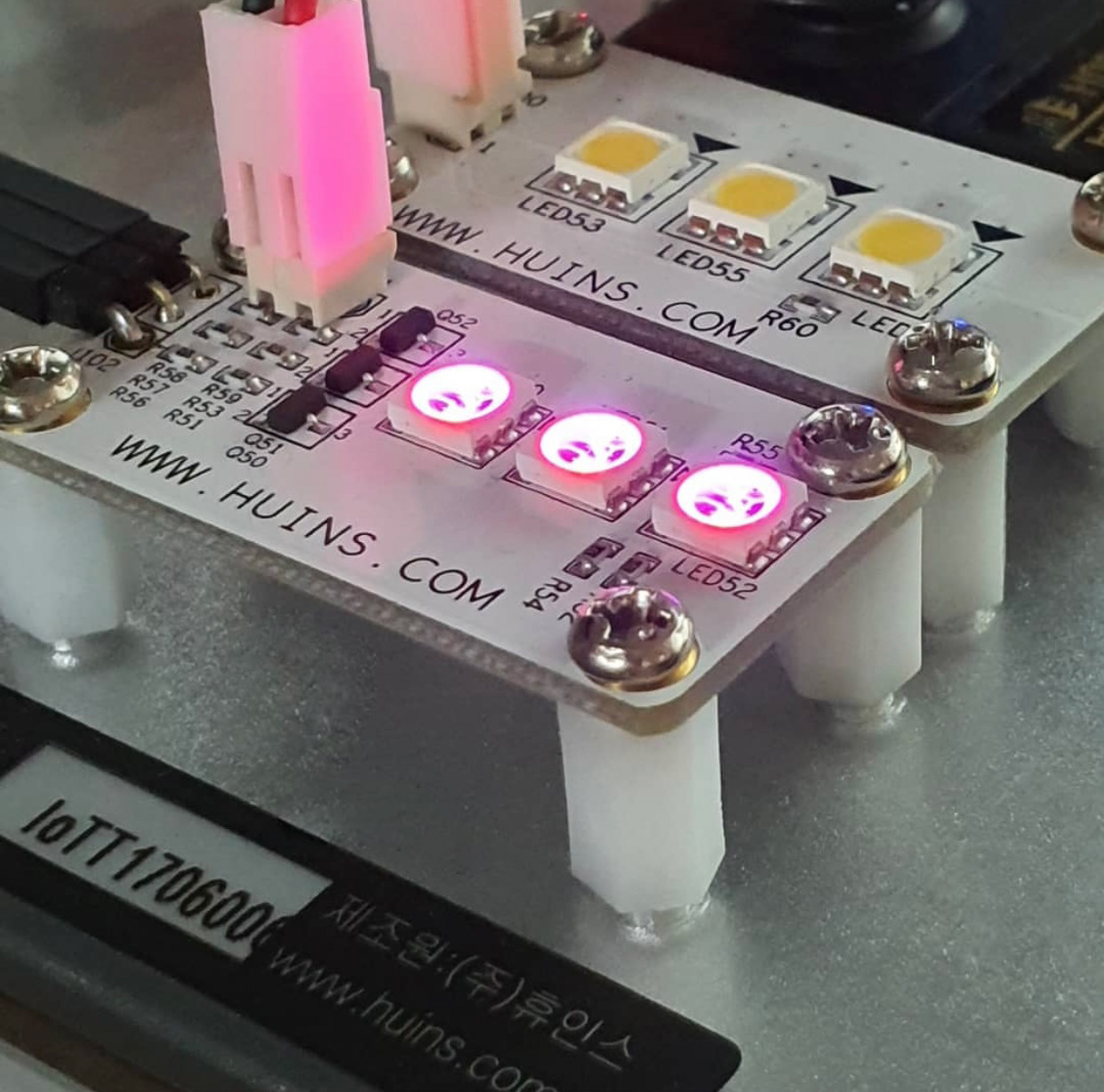
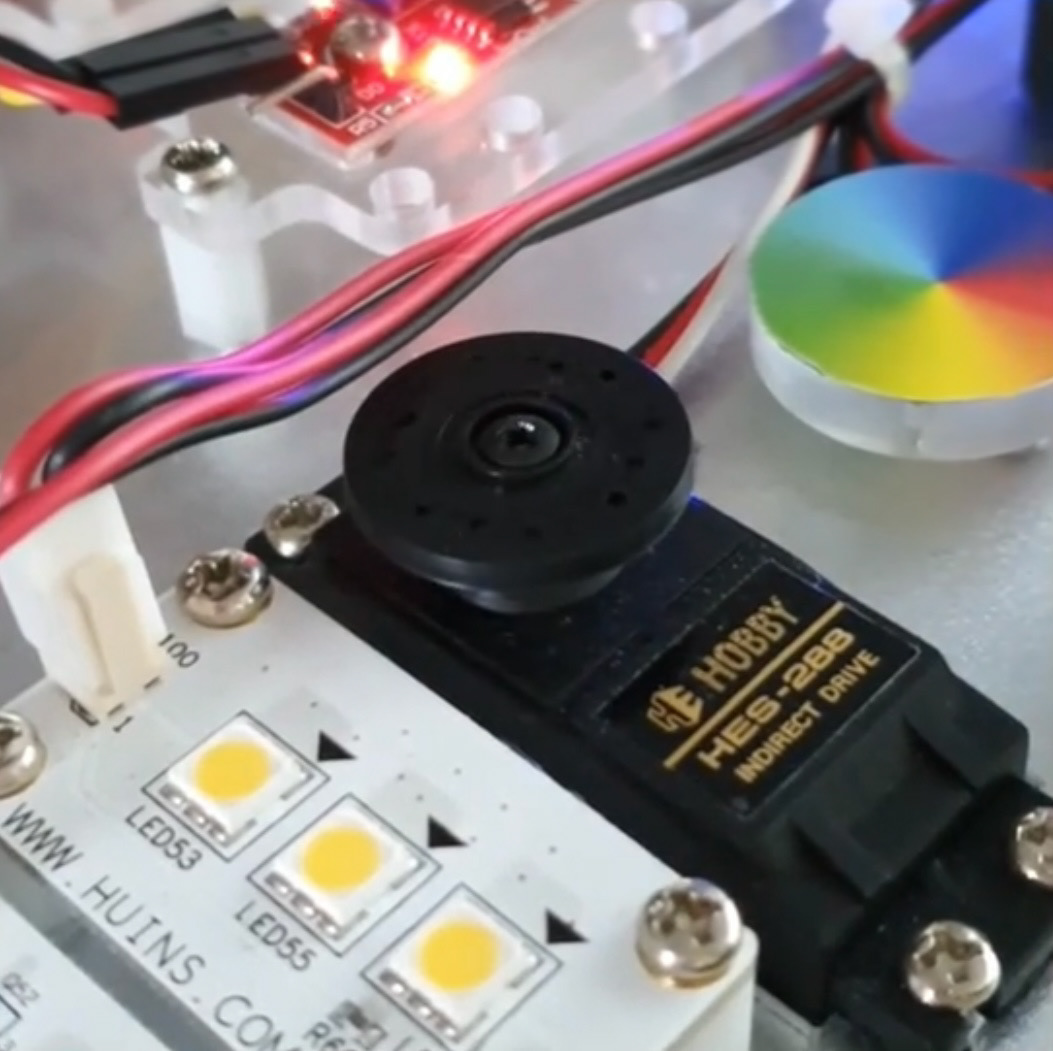
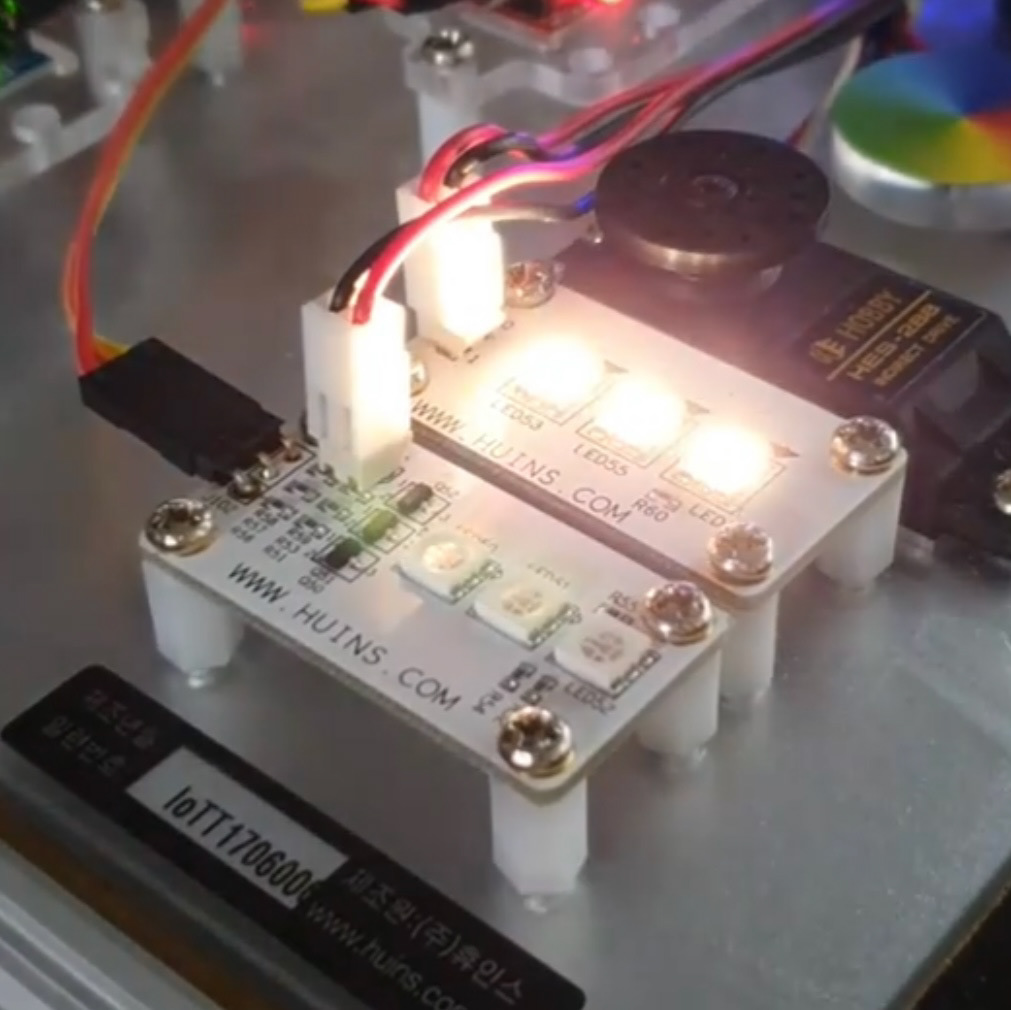
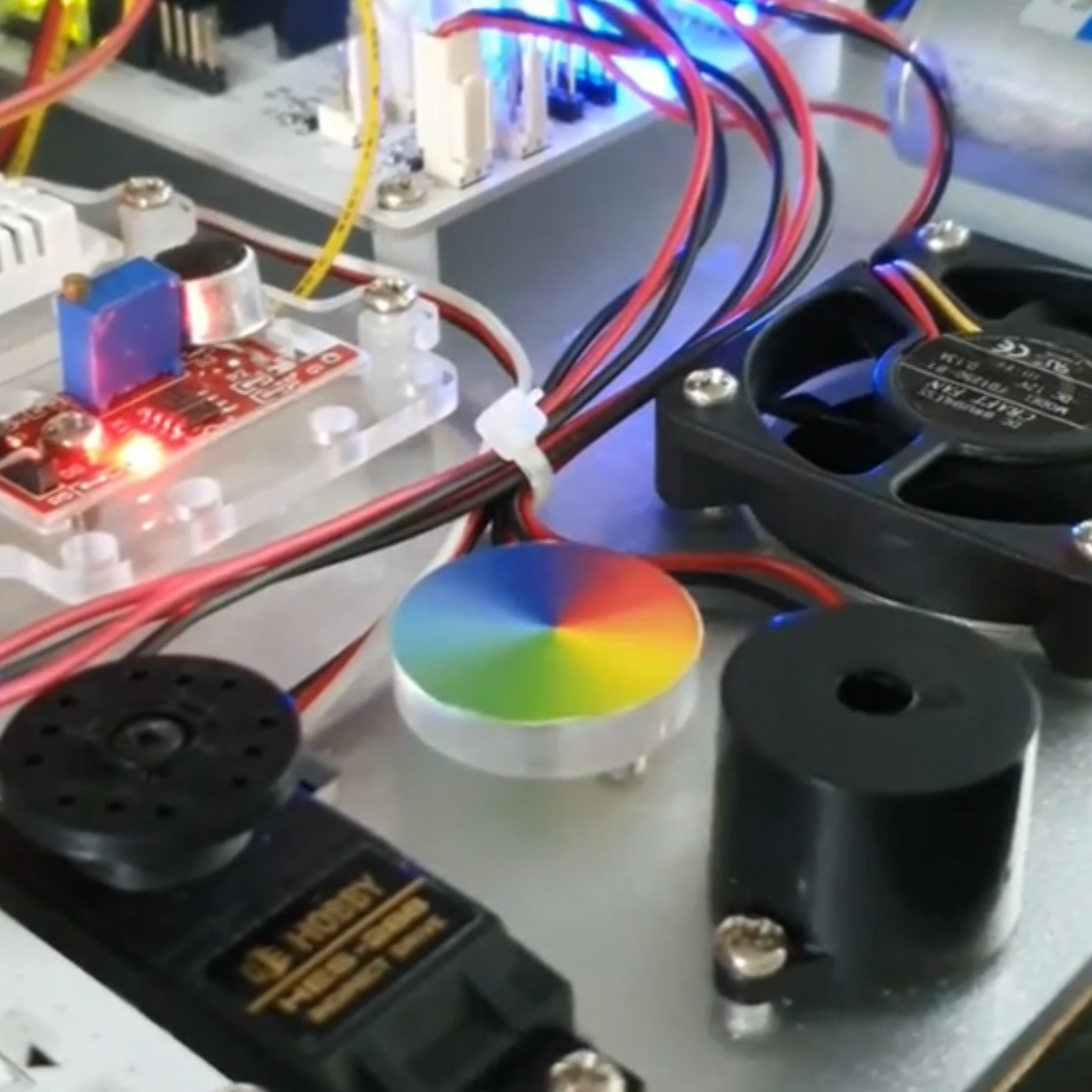
Personal Project / Raspberry Pi Programming in Python
Hongik Univ. Electronic and Electrical Engineering
2019-2 창직IoT종합설계입문
2019.12.
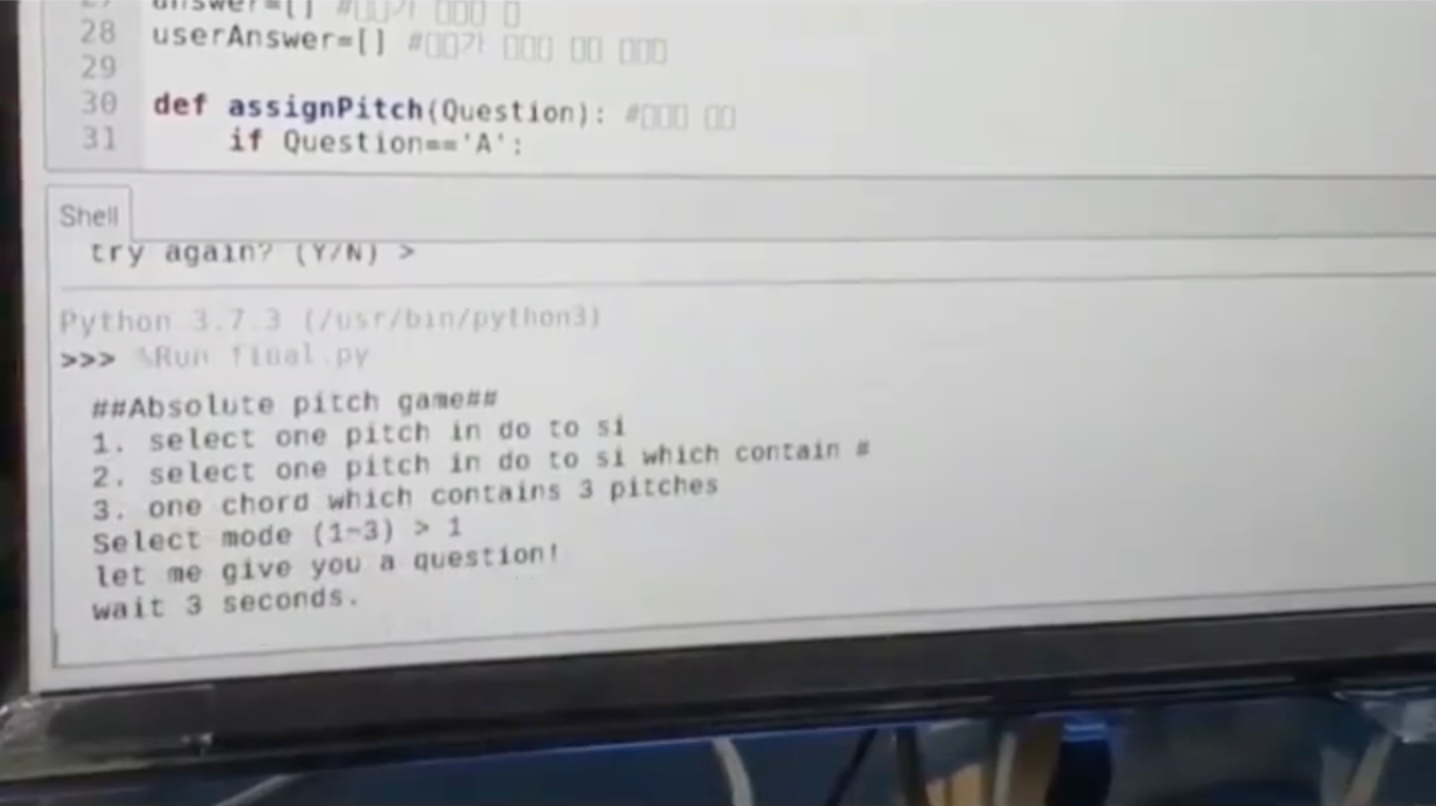
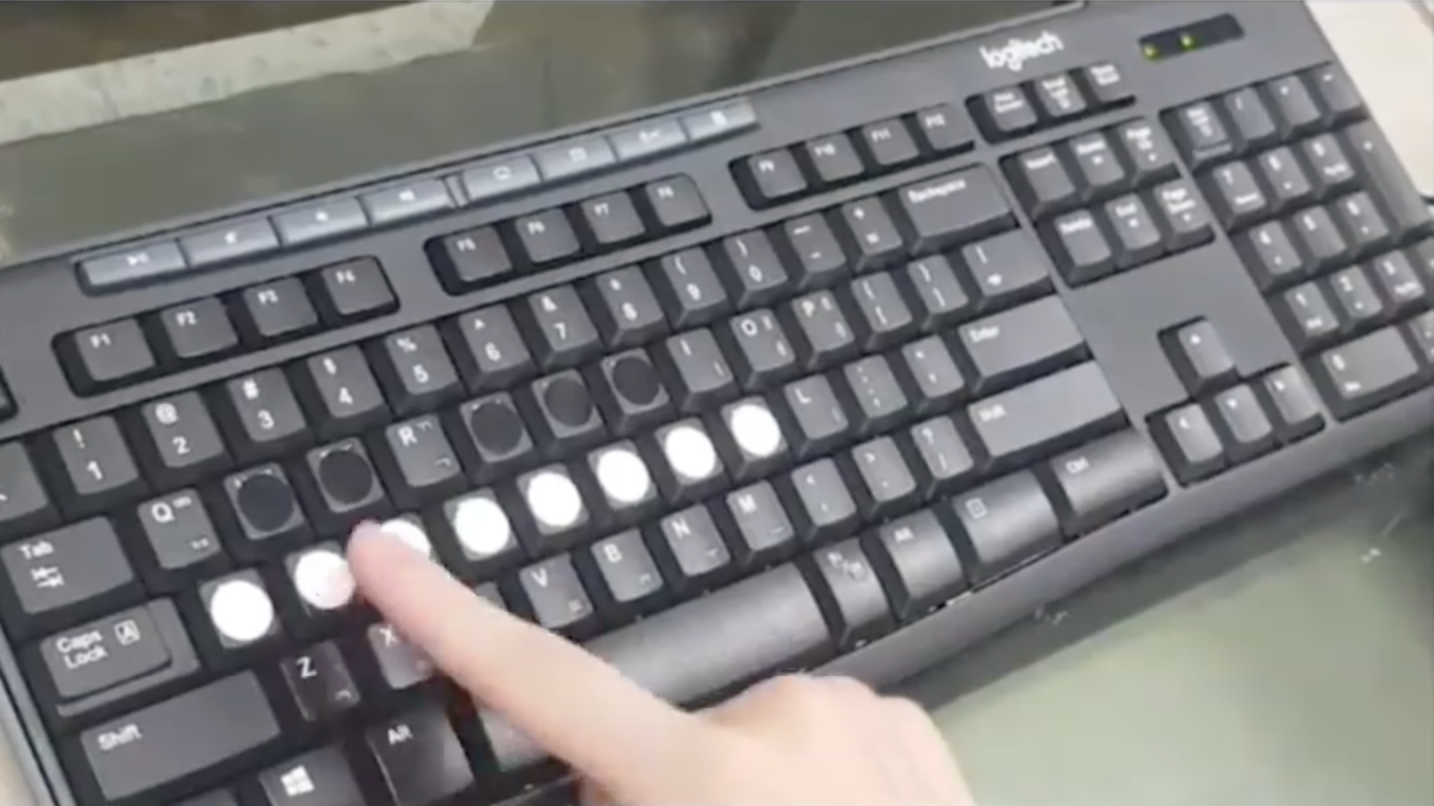
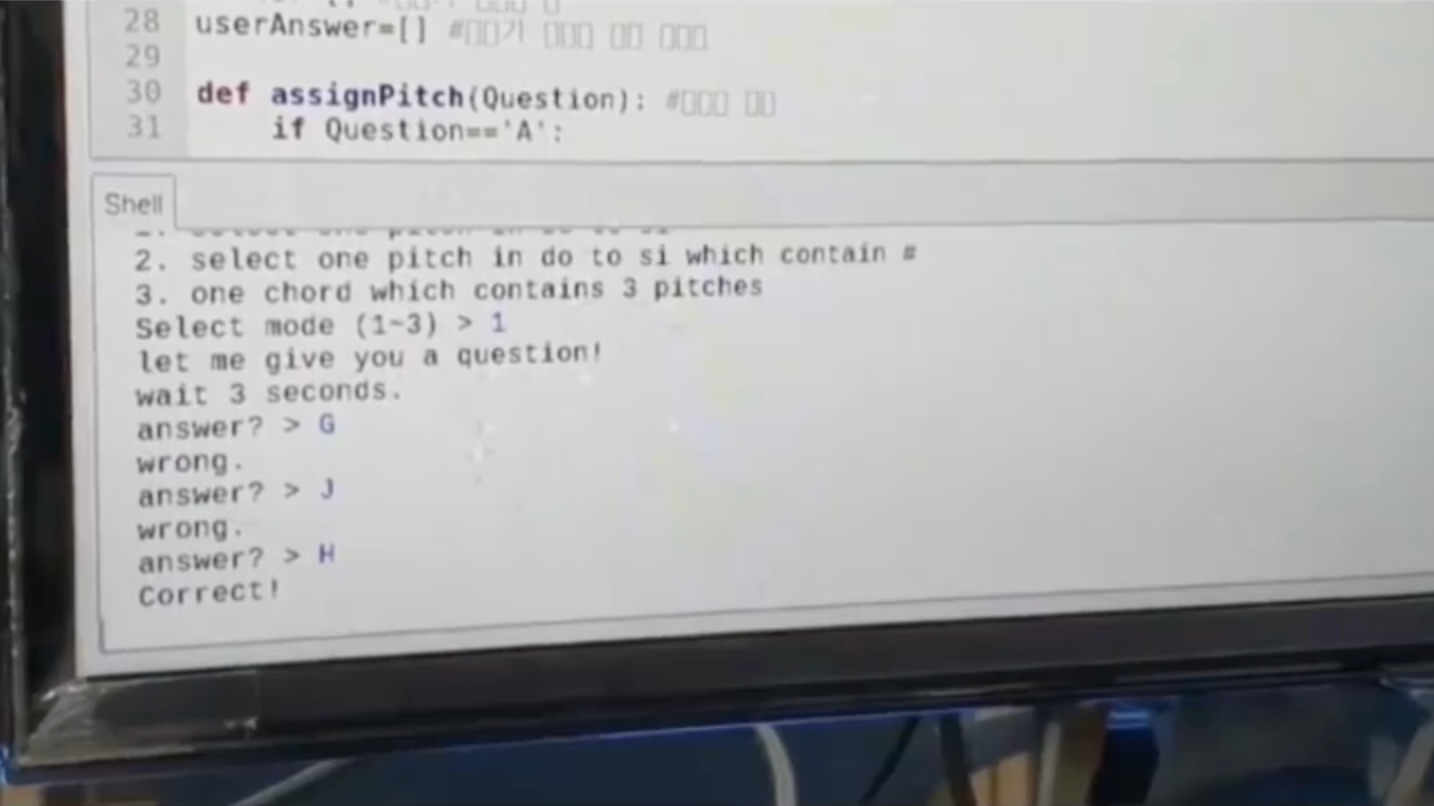
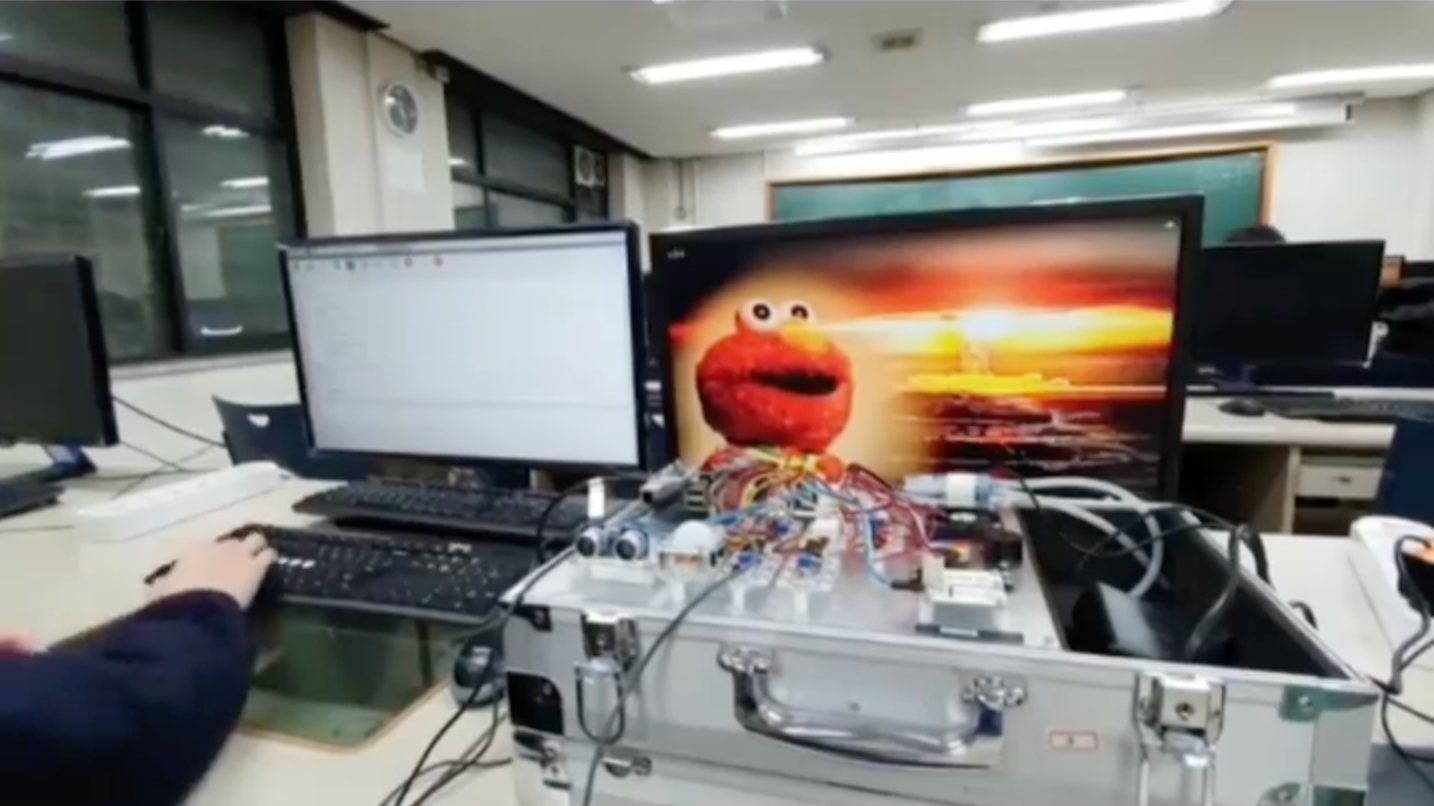
라즈베리파이의 액츄에이터를 활용하여 사물인터넷 시스템을 구현하는 프로젝트다.
Pitch값에 따라 다른 주파수의 소리를 출력하는 부저 액츄에이터를 이용하여 절대음감 게임을 제작했다.
사용자가 고른 모드에 따라 세 가지 방식으로 게임이 진행된다.
첫번째, 도~시 중 한 음계를 랜덤으로 골라 출제하는 모드
두번째, 도~시에 #까지 더해 한 음계를 랜덤으로 골라 출제하는 모드
세번째, 도~시에서 세 음계를 돌라 화음을 출제하는 모드
모드 1~3 중 하나를 선택하면
부저 액츄에이터를 통해 3초 이내에 문제가 출제된다.
틀렸을 경우 출제됐던 소리가 다시 재생되며,
정답일 경우 추가 게임을 시작할 것인지 이대로 종료할 것인지를 묻는다.
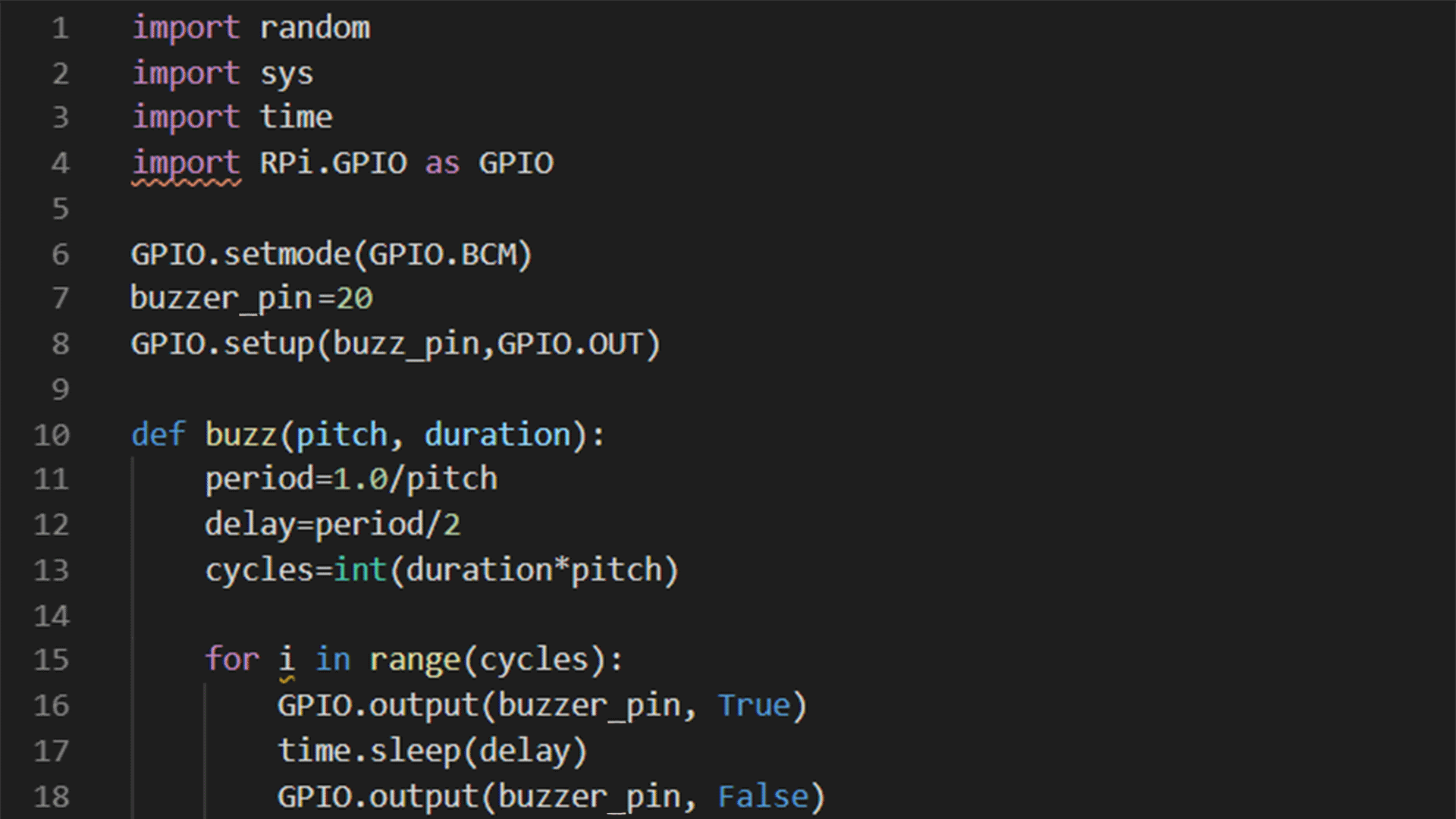
import random
import sys
import time
import RPi.GPIO as GPIO
import sys
import time
import RPi.GPIO as GPIO
GPIO.setmode(GPIO.BCM)
buzz_pin=20
GPIO.setup(buzz_pin,GPIO.OUT)
buzz_pin=20
GPIO.setup(buzz_pin,GPIO.OUT)
def buzz(pitch, duration):
period=1.0/pitch
delay=period/2
cycles=int(duration*pitch)
period=1.0/pitch
delay=period/2
cycles=int(duration*pitch)
for i in range(cycles):
GPIO.output(buzzer_pin, True)
time.sleep(delay)
GPIO.output(buzzer_pin, False)
time.sleep(delay)
GPIO.output(buzzer_pin, True)
time.sleep(delay)
GPIO.output(buzzer_pin, False)
time.sleep(delay)
DoSi=['A','S','D','F','G','H','J'] #도레미파솔라시
DoSiSharp=['A','W','S','E','D','F','T','G','Y','H','U','J'] #샵포함
numberOfAnswers=0 #정답개수 (모드1,2는 1개, 모드3은 3개)
correctAnswer='' #문제(정답)
mode=0 #게임모드 (1~3)
answer=[] #유저가 입력한 답
userAnswer=[] #유저가 입력한 답의 정오답
def assignPitch(Question): #피치수 할당
if Question=='A':
pitch=256.87
elif Question=='W':
pitch=272.6
elif Question=='S':
pitch=288.33
elif Question=='E':
pitch=305.98
elif Question=='D':
pitch=323.63
elif Question=='F':
pitch=332.88
elif Question=='T':
pitch=363.87
elif Question=='G':
pitch=384.87
elif Question=='Y':
pitch=308.435
elif Question=='H':
pitch=432.00
elif Question=='U':
pitch=458.45
else:
pitch=484.90
#print(pitch) #피치 담겨있는 것 확인
return pitch
def correctSound(): #정답딩동댕
print('Correct!')
buzz(assignPitch('A'),0.5)
buzz(assignPitch('D'),0.5)
buzz(assignPitch('G'),0.5)
print('Correct!')
buzz(assignPitch('A'),0.5)
buzz(assignPitch('D'),0.5)
buzz(assignPitch('G'),0.5)
def selectMode(): #모드선택과 정답 할당
print('1. select one pitch in do to si\n2. select one pitch in do to si which contain # \n3. one chord which contains 3 pitches')
mode=input('Select mode (1~3) > ')
print('1. select one pitch in do to si\n2. select one pitch in do to si which contain # \n3. one chord which contains 3 pitches')
mode=input('Select mode (1~3) > ')
global correctAnswer
if mode=='1':
correctAnswer=random.choice(DoSi)
numberOfAnswers=1
elif mode=='2':
correctAnswer=random.choice(DoSiSharp)
numberOfAnswers=1
elif mode=='3':
correctAnswer=''
list_tmp=random.sample(DoSi,3)
for i in range(0,3):
correctAnswer+=list_tmp[i]
numberOfAnswers=3
else:
print('ERROR: you must select one mode (1~3)!')
selectMode()
correctAnswer=random.choice(DoSi)
numberOfAnswers=1
elif mode=='2':
correctAnswer=random.choice(DoSiSharp)
numberOfAnswers=1
elif mode=='3':
correctAnswer=''
list_tmp=random.sample(DoSi,3)
for i in range(0,3):
correctAnswer+=list_tmp[i]
numberOfAnswers=3
else:
print('ERROR: you must select one mode (1~3)!')
selectMode()
print("let me give you a question!")
print("wait 3 seconds.")
time.sleep(3)
#print('number of answers: ',numberOfAnswers) #정답개수 확인
#print('correct answer: ',correctAnswer) #정답 미리 확인
print("wait 3 seconds.")
time.sleep(3)
#print('number of answers: ',numberOfAnswers) #정답개수 확인
#print('correct answer: ',correctAnswer) #정답 미리 확인
def game():
selectMode() #모드 고르기
while(True):
for letter in correctAnswer:
buzz(assignPitch(letter),1) #소리 1초간 들려주기
#print(letter,'를 1초간 재생')
time.sleep(0.5)
answer=input('answer? > ')
if numberOfAnswers==1: #모드 1,2에서
if answer==correctAnswer: #맞히면
correctSound()
restart=input("try again? (Y/N) > ")
if restart=='Y':
game()
else:
sys.exit()
else: #틀리면
print("wrong.")
else: #모드 3에서
if answer==correctAnswer: #맞히면
correctSound()
restart=input("try again? (Y/N) > ")
if restart=='Y':
game()
else:
sys.exit()
else: #틀리면
print("wrong.")
print('##Absolute pitch game##') #타이틀
game()